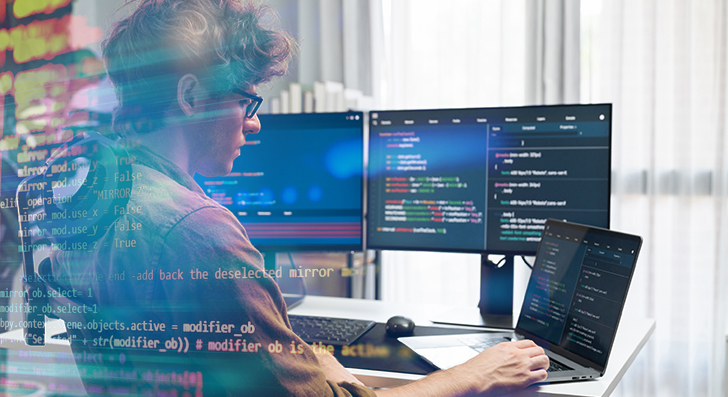
Scalability means your application can manage growth—extra people, a lot more data, and a lot more targeted traffic—without having breaking. As a developer, making with scalability in mind will save time and anxiety later. Below’s a clear and practical tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not one thing you bolt on afterwards—it should be component within your program from the start. Several purposes fail if they develop rapid mainly because the original style and design can’t deal with the additional load. As a developer, you should Imagine early about how your process will behave stressed.
Start out by creating your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular design or microservices. These designs crack your app into smaller sized, impartial pieces. Every module or provider can scale By itself without the need of affecting The full procedure.
Also, consider your database from working day just one. Will it have to have to handle a million consumers or merely a hundred? Select the appropriate style—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Yet another critical place is to stop hardcoding assumptions. Don’t generate code that only is effective less than current conditions. Consider what would occur If the consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that aid scaling, like information queues or party-pushed units. These assistance your application cope with far more requests with no receiving overloaded.
Once you Construct with scalability in mind, you're not just getting ready for success—you might be cutting down foreseeable future head aches. A nicely-planned procedure is simpler to keep up, adapt, and expand. It’s far better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the suitable database is really a key Component of setting up scalable apps. Not all databases are developed the same, and utilizing the Improper one can sluggish you down or perhaps cause failures as your application grows.
Begin by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. They are strong with interactions, transactions, and consistency. In addition they help scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your facts is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and will scale horizontally much more quickly.
Also, consider your go through and generate patterns. Have you been accomplishing plenty of reads with less writes? Use caching and browse replicas. Have you been dealing with a major create load? Investigate databases which will handle large publish throughput, or simply event-based mostly facts storage techniques like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not want State-of-the-art scaling options now, but deciding on a databases that supports them means you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access patterns. And always monitor database efficiency while you expand.
In a nutshell, the best database is determined by your app’s structure, speed requires, And exactly how you anticipate it to grow. Take time to select sensibly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every compact hold off adds up. Poorly written code or unoptimized queries can decelerate functionality and overload your technique. That’s why it’s vital that you Develop economical logic from the beginning.
Commence by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular functions. Keep your capabilities quick, focused, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish matters down a lot more than the code alone. Be sure each question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, especially across substantial tables.
Should you see exactly the same knowledge remaining requested over and over, use caching. Retail store the results briefly working with applications like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your databases functions after you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done good with 100 information may possibly crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when necessary. These methods assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more traffic. If everything goes through 1 server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools assistance keep the application rapidly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of one server doing many of the get the job done, the load balancer routes end users to distinct servers depending on availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-centered solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it from the databases each and every time. You can provide it in the cache.
There's two frequent different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Shopper-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database load, increases speed, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And constantly make sure your cache is up to date when information does transform.
In short, load balancing and caching are basic but powerful resources. Jointly, they assist your app take care of extra customers, keep speedy, and recover from troubles. If you propose to grow, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t should invest in components or guess future capacity. When traffic increases, you are able to include a lot more sources with only a few clicks or instantly working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention website to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and every thing it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it routinely.
Containers also make it straightforward to independent aspects of your app into services. You could update or scale areas independently, which is perfect for overall performance and trustworthiness.
Briefly, utilizing cloud and container applications implies you could scale rapidly, deploy easily, and Recuperate immediately when difficulties materialize. If you need your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease chance, and help you keep focused on creating, not repairing.
Monitor Every little thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make improved decisions as your app grows. It’s a essential Component of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for users to load pages, how frequently mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a limit or simply a company goes down, you'll want to get notified immediately. This allows you take care of difficulties rapidly, typically just before customers even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a brand new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and details enhance. With out checking, you’ll overlook indications of difficulties until finally it’s too late. But with the best equipment in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and making sure it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications need a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the correct instruments, you are able to Create applications that develop efficiently without breaking under pressure. Start out small, Consider significant, and Develop sensible.